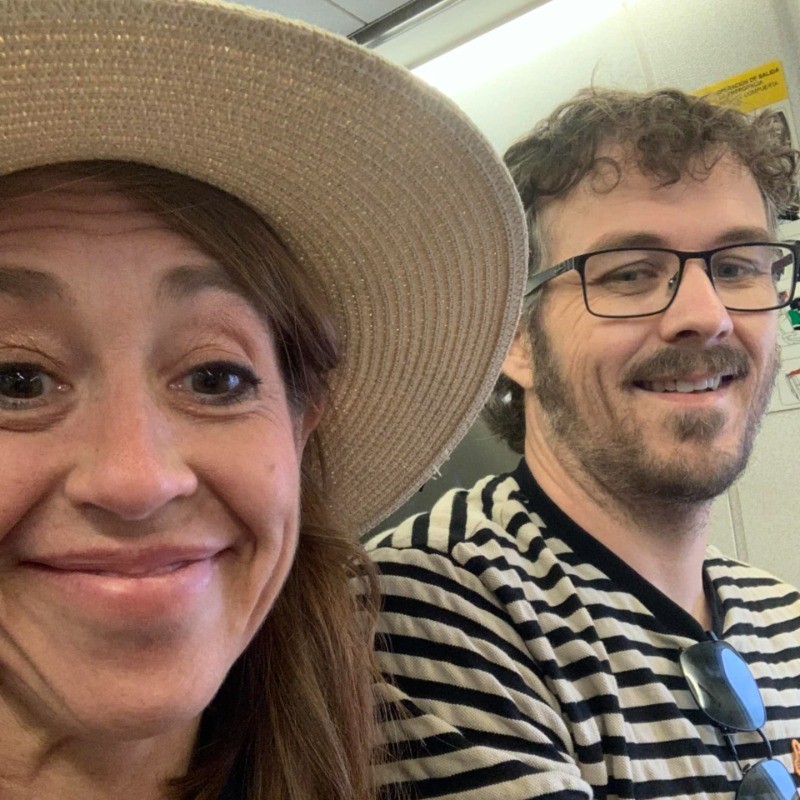
Authentication: Setting Up Supabase and Svelte/SvelteKit for Server-Side Rendering
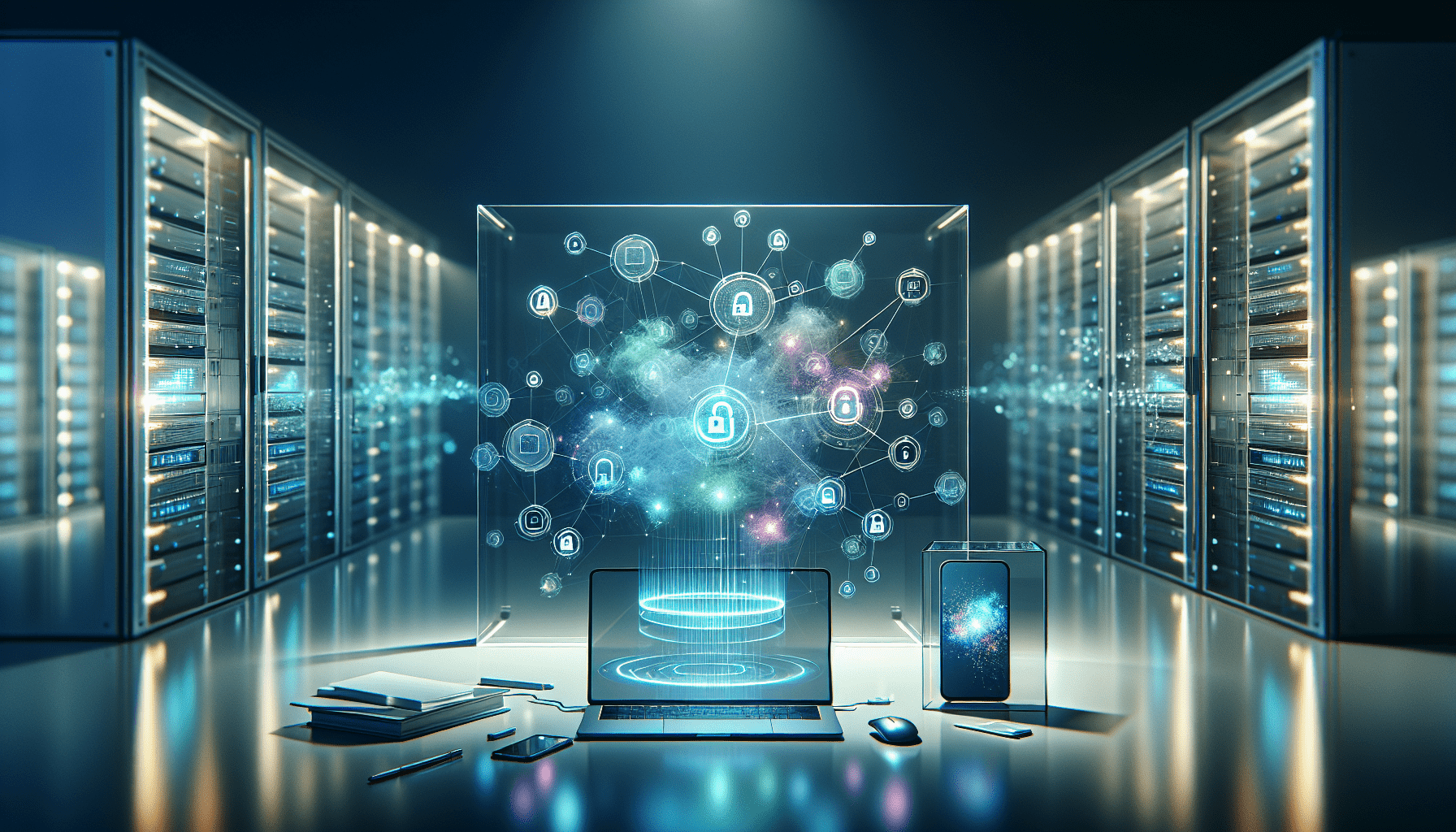
Setting up Supabase for Server-Side Rendering in SvelteKit
This article delves into the process of setting up Supabase for server-side rendering in SvelteKit. It explores the integration of SvelteKit and Supabase, guiding readers through the configuration of the Supabase client for server-side use. The article also covers the creation of authentication pages and concludes with insights on implementing Svelte Supabase auth effectively. By the end, developers will have a solid understanding of how to build secure and performant authentication systems using these cutting-edge technologies.
Understanding SvelteKit and Supabase Integration
SvelteKit and Supabase integration offers developers a powerful combination for building modern web applications with robust authentication capabilities. This integration allows for the implementation of server-side rendering (SSR) and efficient user management.
To begin the integration process, developers need to install the necessary packages. The @supabase/supabase-js package provides the core functionality for interacting with Supabase, while the @supabase/ssr package offers helper functions specifically designed for server-side rendering environments.
Benefits of Server-Side Rendering
Server-side rendering in SvelteKit provides several advantages when integrated with Supabase:
- Enhanced Security: SSR allows for cookie-based authentication, which is more secure than client-side token storage.
- Improved Performance: By rendering pages on the server, initial load times can be reduced, leading to a better user experience.
- SEO Optimization: Server-rendered content is more easily indexable by search engines, potentially improving search rankings.
- Seamless Authentication Flow: The integration enables the creation of a Supabase client that works across the entire SvelteKit stack, including pages, layouts, server, and hooks.
Supabase Authentication Features
Supabase offers a range of authentication features that can be leveraged in a SvelteKit application:
- Magic Link Authentication: Developers can implement a secure login/signup process using magic links.
- Session Management: The integration allows for efficient handling of user sessions throughout the application.
- User Profile Management: Supabase enables easy storage and retrieval of user profile information.
- File Storage: The integration supports file uploads, such as profile photos, using Supabase Storage.
Configuring Supabase Client for Server-Side Use
Creating a Supabase Client
To configure the Supabase client for server-side use in SvelteKit, developers need to set up server-side hooks in the src/hooks.server.js
file. These hooks create a request-specific Supabase client using the user credentials from the request cookie, which is then used for server-only code.
Here’s an example of how to initialize the client on the server:
import { PUBLIC_SUPABASE_URL, PUBLIC_SUPABASE_ANON_KEY } from '$env/static/public';
import { createServerClient } from '@supabase/ssr';
export const handle = async ({ event, resolve }) => {
event.locals.supabase = createServerClient(PUBLIC_SUPABASE_URL, PUBLIC_SUPABASE_ANON_KEY, {
getAll: () => event.cookies.getAll(),
setAll: (cookiesToSet) => {
cookiesToSet.forEach(({ name, value, options }) => {
event.cookies.set(name, value, { ...options, path: '/' });
});
},
});
event.locals.safeGetSession = async () => {
const { session, user } = await event.locals.supabase.auth.getSession();
return { session, user };
};
return resolve(event, {
filterSerializedResponseHeaders(name) {
return name === 'content-range' || name === 'x-supabase-api-version';
},
});
};
This code sets up the Supabase client with the necessary configuration for server-side rendering, including handling cookies and session management.
Handling Auth Events
To handle authentication events on the client-side, developers should set up a listener for Auth events. This listener helps manage session refreshes and signouts. It’s crucial to implement this listener to ensure seamless authentication flow throughout the application.
Building Authentication Pages
Login Page Implementation
To implement a login page using SvelteKit and Supabase, developers can create a form that collects the user’s email and password. The login functionality can be implemented using the signInWithPassword()
method provided by Supabase. Here’s an example of how to create a basic login form using Svelte 5:
<script>
import { supabase } from '$lib/supabaseClient';
let email = $state('');
let password = $state('');
async function login(event) {
event.preventDefault();
const { data, error } = await supabase.auth.signInWithPassword({ email, password });
if (error) {
console.error('Error:', error.message);
} else {
console.log('Logged in:', data);
// Redirect or update UI as needed
}
}
</script>
<form action={login}>
<div>
<label for="email">Email address</label>
<input type="email" id="email" name="email" bind:value={email} required />
</div>
<div>
<label for="password">Password</label>
<input type="password" id="password" name="password" bind:value={password} required />
</div>
<button type="submit">Sign In</button>
</form>
Signup and Email Confirmation
For the signup process, developers can create a similar form that collects the user’s email and password. The signUp()
method from Supabase can be used to handle the signup process. If email confirmations are enabled, Supabase will send a confirmation email to the user.
To enable email confirmations, developers need to configure the email settings in the Supabase dashboard or the configuration file for self-hosted projects. The confirmation email template can be customized to include a token hash for server-side authentication:
{{ .SiteURL }}/auth/confirm?token_hash={{ .TokenHash }}&type=email
After the user clicks the confirmation link, a server endpoint needs to be created to handle the token exchange:
// src/routes/auth/confirm/+server.js
import { redirect } from '@sveltejs/kit';
export const GET = async ({ url, locals: { supabase } }) => {
const token_hash = url.searchParams.get('token_hash');
const type = url.searchParams.get('type');
const next = url.searchParams.get('next') ?? '/account';
if (token_hash && type) {
const { error } = await supabase.auth.verifyOtp({ type, token_hash });
if (!error) {
redirect(303, next);
}
}
// Handle errors
redirect(303, '/auth/error');
};
Password Reset Functionality
To implement password reset functionality, developers need to create two pages: a reset password page and a change password page.
Reset Password Page:
- Create a publicly accessible page where users can enter their email address.
- Use the
supabase.auth.resetPasswordForEmail()
method to send a password reset email.
Change Password Page:
- Create a page accessible only to authenticated users.
- Implement a form to collect the new password.
- Use the
supabase.auth.updateUser()
method to update the user’s password.
Here’s an example of how to implement the password reset functionality using Svelte 5:
<script>
import { supabase } from '$lib/supabaseClient';
let email = $state('');
let message = $state('');
async function resetPassword(event) {
event.preventDefault();
const { error } = await supabase.auth.resetPasswordForEmail(email, {
redirectTo: 'http://localhost:3000/change-password',
});
if (error) {
message = 'Error: ' + error.message;
} else {
message = 'Password reset email sent. Check your inbox.';
}
}
</script>
<form action={resetPassword}>
<input type="email" bind:value={email} placeholder="Enter your email" required />
<button type="submit">Reset Password</button>
</form>
{#if message}
<p>{message}</p>
{/if}
By implementing these authentication pages, developers can create a secure and user-friendly authentication system using SvelteKit and Supabase.
Conclusion
The integration of SvelteKit and Supabase offers developers a powerful toolkit to build secure and efficient web applications with robust authentication systems. This combination enables seamless server-side rendering, enhancing security and performance while providing a user-friendly experience. The setup process, including configuring the Supabase client for server-side use and implementing authentication pages, lays a strong foundation for creating modern, scalable web applications.
As web development continues to evolve, the SvelteKit-Supabase duo stands out as a versatile solution for developers looking to create cutting-edge applications. By leveraging the strengths of both technologies, developers can craft secure, performant, and feature-rich web experiences. This approach not only streamlines the development process but also paves the way for future innovations in web application design and functionality.
FAQs
Does Svelte support server-side rendering? Server-side rendering (SSR) is a technique where HTML is generated on the server rather than in the browser. SvelteKit, by default, employs this method for rendering web pages.
Is Supabase designed to operate on the client-side or server-side? Supabase can be utilized on the server-side through the use of the
createServerClient
function, which is particularly handy in route loaders and route actions.Does Supabase offer user authentication features? Yes, Supabase includes an authentication system that supports Time-based One-Time Password (TOTP) multi-factor authentication (MFA). This type of MFA generates a temporary password via an authenticator app controlled by the user and is enabled by default in all Supabase projects.
How can one integrate Supabase with a SvelteKit application? To use Supabase with SvelteKit, start by creating a SvelteKit application using the npm create command. Next, install the Supabase client library, which is designed to facilitate interaction with Supabase services within a SvelteKit app. Finally, proceed to start your application.
References
[1] - https://supabase.com/docs/guides/getting-started/tutorials/with-sveltekit
[2] - https://supabase.com/docs/guides/auth/auth-helpers/sveltekit
[3] - https://supabase.com/docs/guides/getting-started/quickstarts/sveltekit
[4] - https://dev.to/kvetoslavnovak/supabase-ssr-auth-48j4
[5] - https://supabase.com/docs/guides/auth/server-side/creating-a-client
[6] - https://supabase.com/docs/reference/javascript/typescript-support
[7] - https://supabase.com/docs/guides/api/rest/generating-types